Making your app modular: Yapsy
That a plugin architecture for a complex app is a good idea is one of those things that most people kinda agree on. One thing we don't quite agree is how the heck are we going to make out app modular?
One way to do it (if you are coding python) is using Yapsy.
Yapsy is awesome. Also, yapsy is a bit underdocumented. Let's see if this post fixes that a bit and leaves just the awesome.
Update: I had not seen the new Yapsy docs, released a few days ago. They are much better than what was there before :-)
Here's the general idea behind yapsy:
You create a Plugin Manager that can find and load plugins from a list of places (for example, from ["/usr/share/appname/plugins", "~/.appname/plugins"]).
A plugin category is a class.
There is a mapping between category names and category classes.
-
A plugin is a module and a metadata file. The module defines a class that inherits from a category class, and belongs to that category.
The metadata file has stuff like the plugin's name, description, URL, version, etc.
One of the great things about Yapsy is that it doesn't specify too much. A plugin will be just a python object, you can put whatever you want there, or you can narrow it down by specifying the category class.
In fact, the way I have been doing the category classes is:
Start with an empty class
Implement two plugins of that category
If there is a chunk that's much alike in both, move it into the category class.
But trust me, this will all be clearer with an example :-)
I will be doing it with a graphical PyQt app, but Yapsy works just as well for headless of CLI apps.
Let's start with a simple app: an HTML editor with a preview widget.
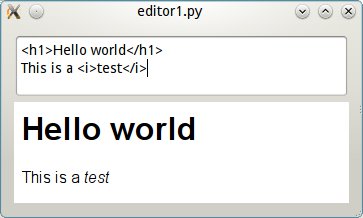
A simple editor with preview
Here's the code for the app, which is really simple (it doesn't save or do anything, really, it's just an example):
But this application has an obvious limit: you have to type HTML in it. Why not type python code in it and have it convert to HTML for display? Or Wiki markup, or restructured text?
You could, in principle, just implement all those modes, but then you are assuming the responsability of supporting every thing-that-can-be-turned-into-HTML. Your app would be a monolith. That's where yapsy enters the scene.
So, let's create a plugin category, called "Formatter" which takes plain text and returns HTML. Then we add stuff in the UI so the user can choose what formatter he wants, and implement two of those.
Here's our plugin category class:
Of course what good is a plugin architecture without any plugins for it? So, let's create two plugins.
First: a plugin that takes python code and returns HTML, thanks to pygments.
See how it goes into a plugins folder? Later on we will tell yapsy to search there for plugins.
To be recognized as a plugin, it needs a metadata file, too:
And really, that's all there is to making a plugin. Here's another one for comparison, which uses docutils to format reStructured Text:
And here they are in action:
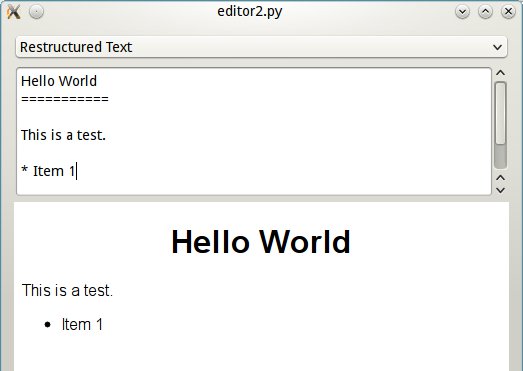
reSt mode
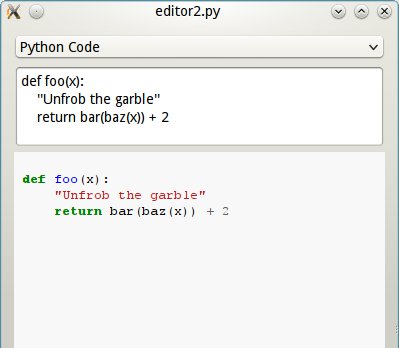
Python mode
Of course using categories you can do things like a "Tools" category, where the plugins get added to a Tools menu, too.
And here's the application code:
In short: this is easy to do, and it leads to fixing your application's internal structure, so it helps you write better code.
Perhaps worth reusing http://github.com/ActiveSta... for coming up with plugin dir in cross-platform way?
Yes, usually ~/.appname/plugins and "whereverthe.py_is_installed/plugins" are good enough, but that's probably a better idea.
Thanks for this great walk-through and presentation of yapsy!
I'm yapsy's maintainer and though I tried to improve the doc recently I reckon this kind of tutorial is really the best explanation of how and why yapsy can be used.
Thanks again and all the best for your own projects !
Thanks to you for yapsy, it has been a great help in the project where I'm using it :-)
Great!
Just a little bit:
Shouldn't editor2.py contain a line to import formatter, something like "from categories import Formatter"
Without that, line 22 throws, otherwise, it works great.
Yup, real bug. I'll fix it ASAP.
I can't seem to get the example to run. When I execute:
python editor2.py
The interface comes up, but the only option in the Formatters widget is None. Also, the line:
print self.manager.getPluginsOfCategory("Formatters")
just prints out "[]"
Could I be doing something wrong? I'm on Ubuntu 10.10 with python version 2.6.6 and yapsy version 1.8
Thanks,
dan
Nevermind. It turns out that I just didn't have the dependencies installed for the plugins (e.g., pygments), and so I guess the plugin loader was just faiing silently.
If you change the logging level to DEBUG you will get the errors for the plugins.
Something like
import logging
logging.basicConfig(level=logging.DEBUG)
Thanks a lot, that’s very helpful. I can’t imagine how it was before the “new, much better Yapsy docs” were released. It’s ridiculous, they don’t even have an example that shows what to do twith the plugins you write, just how to load them.
this is really interesting viewpoint on the subject i might add
Man ... Beautiful . Amazing ... I will bookmark your website and use the your RSS feed also
Hello, Thanks for this tut.
I'm searching for a complete example of using YAPSY for extending component GUI (menu, windows,..) of a PySide/pyQT app.
Do you have any sample explicit for me ?
Regards,